Responsive web design ensures websites adapt seamlessly across devices, from smartphones to desktops. This lesson explores the essential breakpoints for desktops, tablets, and phones, guiding the creation of adaptable layouts with Tailwind CSS to enhance user experience on any screen.
What are breakpoints?
Breakpoints in web design are specific screen widths (or other conditions) that trigger changes in the layout and appearance of a website to ensure it displays optimally across different devices. They are a fundamental part of responsive design, allowing content to adapt to the varying screen sizes of desktops, tablets, and smartphones.
By using CSS media queries or using Tailwind classes, designers can apply different styles based on these breakpoints, making websites flexible and user-friendly no matter the device used for viewing. Essentially, breakpoints help create a seamless and accessible web experience for all users, regardless of how they access the internet.
Lets look at the common breakpoints considered for website design:
Desktops and Large Monitors
- Breakpoint: > 1024 pixels wide.
- Encompasses large screens suited for desktops and monitors.
- Resolutions range from 1280 pixels to 4K and beyond.
- Design for a minimum width of 1024 pixels, accommodating higher resolutions for contemporary monitors.
Tablets (and Large Phones in Landscape)
- Breakpoint: 768 to 1024 pixels wide.
- Covers intermediate screens, including tablets and large smartphones in landscape orientation.
- Utilized in both portrait and landscape modes.
- Key design considerations involve touch interaction and adaptable layouts for varying aspect ratios.
Large Phones - Landscape Mode/Small Tablets
- Breakpoint: 640 to 768 pixels wide.
- Targets large smartphones in landscape mode and smaller tablets.
- Focus on optimizing for touch controls and ensuring content is legible without excessive zooming.
- Responsive adjustments may include stacking elements vertically or simplifying layouts.
Mobile Phones in Portrait Mode
- Breakpoint: < 640 pixels wide.
- Primarily for smartphones held in portrait orientation.
- Design principles prioritize ease of navigation with one hand, readable text sizes, and accessible touch targets.
- Consideration for vertical space efficiency is key, given the limited width.
Responsive Design in Tailwind
Tailwind CSS adopts a mobile-first design approach, providing a set of breakpoints that cater to various device sizes. This methodology emphasizes starting the design process from the smallest screens (mobile devices) and progressively enhancing the design for larger screens (tablets, desktops, etc.) using Tailwind's responsive utility classes. Here's how Tailwind's breakpoints are structured:
- sm: 640px — @media (min-width: 640px) { ... } targets large phones (in landscape mode) and mini-tablets.
- md: 768px — @media (min-width: 768px) { ... } is designed for tablets and above, ensuring content is optimized for intermediate screen sizes.
- lg: 1024px — @media (min-width: 1024px) { ... } aims at desktops and larger tablets, allowing for adjustments suitable for wider screens.
- xl: 1280px — @media (min-width: 1280px) { ... } caters to large desktops, providing additional space for more complex layouts or detailed content.
- 2xl: 1536px — @media (min-width: 1536px) { ... } is intended for very large desktops, offering the opportunity to enhance designs for expansive displays.
Understanding Mobile-First Design
Mobile-first design means crafting your website's styling starting with mobile devices and then applying or overriding those styles for larger devices using breakpoints.
Assume we have a <div> element with a purple background intended for mobile screens:
<div class="bg-purple-500">...</div>
To modify the background color for tablets and larger devices, we use the md: breakpoint modifier:
<div class="bg-purple-500 md:bg-cyan-500">...</div>
- On mobile devices, the background color is purple (bg-purple-500).
- On tablets and devices wider than the md breakpoint (768 pixels), the background color changes to cyan (md:bg-cyan-500). This style then applies to all larger devices unless further overridden at a higher breakpoint.
This methodology ensures that your web design is responsive and adaptive, starting with the most restrictive environment (mobile) and progressively enhancing the experience as the available space increases. Mobile-first design emphasizes usability and performance on mobile devices, aligning with the current trends where mobile browsing predominates.
Example: Responsive Grid Layout Requirement
We aim to design a versatile and responsive grid layout that dynamically adapts to various device sizes, ensuring optimal content display and user experience across all platforms. Our goal is to create a layout that meets the following criteria:
- Desktops and Larger Devices (>1024px): 4 columns gridThe grid should display four columns to make efficient use of the ample screen space available on desktop monitors and larger devices. This layout will cater to users accessing the site on traditional desktop setups and larger screens, providing a comprehensive view of the data.
- Tablets (768px to 1024px): 2 columns gridFor tablet users, who require a balance between readability and efficient use of space, the grid will adjust to two columns. This ensures that content remains accessible without overwhelming the user with too much information at once, suitable for both portrait and landscape orientations.
- Large Phones in Landscape Mode and Mini-Tablets: 2 columns gridRecognizing the versatility of large smartphones and mini-tablets, especially when used in landscape orientation, the layout will also feature two columns. This adaptation maintains usability and content visibility, accommodating the intermediate screen size that bridges mobile phones and full-sized tablets.
- Phones in Portrait Mode: 1 column gridFor the most compact display scenario—phones in portrait mode—the grid will simplify to a single column. This approach prioritizes clarity and ease of navigation for mobile users, ensuring that content is presented in a straightforward, scrollable format without the need for horizontal scrolling or zooming.
Now, let's dive into development:
Step 1: Initial Setup for Mobile Phones (Portrait Mode)
We begin by defining a grid layout that defaults to 1 column for mobile phones, ensuring all items are stacked vertically for easy readability on small screens.
<div class="grid grid-cols-1 gap-4"> <div className="flex justify-center items-center rounded bg-purple-300 h-20">Item 1</div> <div className="flex justify-center items-center rounded bg-cyan-300 h-20">Item 2</div> <div className="flex justify-center items-center rounded bg-pink-300 h-20">Item 3</div> <div className="flex justify-center items-center rounded bg-orange-300 h-20">Item 4</div></div>
Step 2: Adjusting for Large Phones/Mini-Tablets (Landscape Mode)
To accommodate large phones or mini-tablets in landscape mode, we introduce a breakpoint using sm: (for screens =640px wide) to transition to a 2-column layout. This improves the use of space and maintains usability without overcrowding the screen.
<div className="grid grid-cols-1 gap-4 sm:grid-cols-2"> <!-- Grid items --></div>
Note: It's important to note a key distinction in breakpoint nomenclature when transitioning from other frameworks to Tailwind CSS. In Tailwind, the sm breakpoint is designed for large phones or mini-tablets, marking a departure from some frameworks where sm might refer exclusively to standard mobile phones in portrait mode. Consequently, for standard phones like an iPhone in portrait orientation, Tailwind does not require a specific breakpoint modifier, as the mobile-first design principle applies the base styles by default.
Step 3: Maintaining Layout for Tablets
For tablets, which typically start at widths of 768px (md: breakpoint), we aim to keep the 2-column layout. However, Tailwind's mobile-first approach means styles applied at a smaller breakpoint (like sm:) naturally carry over to larger screens until explicitly overridden. Therefore, specifying md:grid-cols-2 is unnecessary if the design from the sm: breakpoint remains unchanged.
Tablet
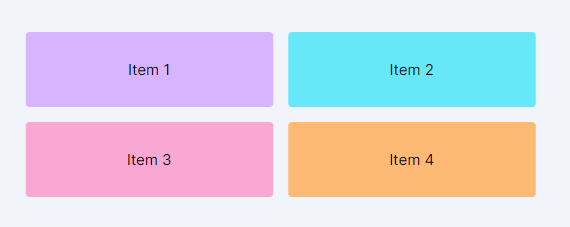
Desktop
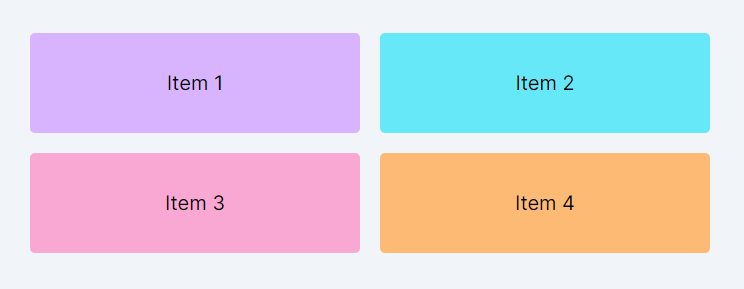
Step 4: Expanding to Desktops and Larger Devices
Finally, for desktops and larger devices (=1024px, using the lg: breakpoint), we expand the grid to display 4 columns, making full use of the wider screen real estate.
<div className="grid grid-cols-1 gap-4 sm:grid-cols-2 lg:grid-cols-4"> <!-- Grid items --></div>
Tablet
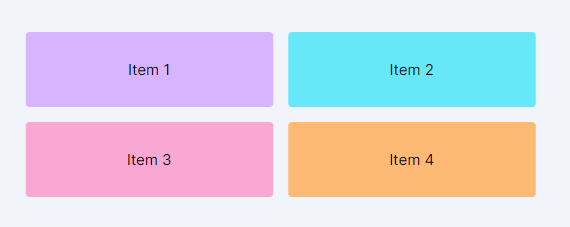
Desktop

In the preceding example, you observed our process of initially crafting a design tailored for mobile devices and gradually adapting it for tablets and desktops. This is the general approach we will follow throughout this course, guiding you in creating responsive designs with a mobile-first perspective.
In this lesson, we've delved into various device sizes, explored Tailwind breakpoints, and gained insight into the concept of mobile-first design through an illustrative example. It's crucial to grasp these foundational concepts before progressing to subsequent lessons in this course. We recommend revisiting this lesson multiple times if any uncertainties arise.
Additionally, for those new to web design, we suggest jotting down the Tailwind breakpoints on a sheet of paper and placing it on your workspace. This handy reference will prove invaluable whenever you encounter questions regarding breakpoints.
In this module, we will delve into a variety of responsive design patterns essential for developing efficient and user-centric web experiences. These patterns tackle distinct challenges and prospects within responsive design, providing approaches and techniques to build websites that are visually attractive, fully functional, and easily accessible.